PHP, Howto capture a Website Screenshot
How to Take Screenshot Captures of Any Website.
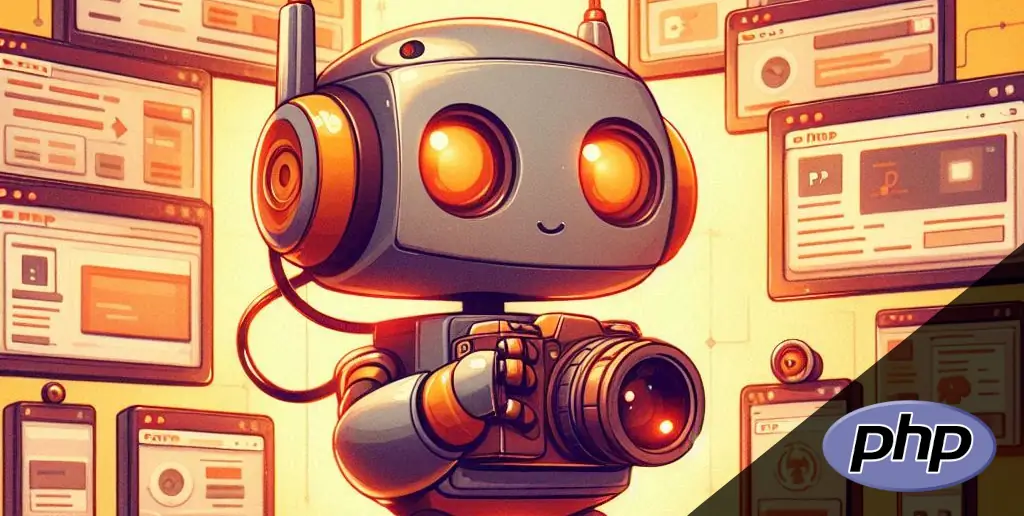
Introduction to Page Capture
In this tutorial, we will see how we can use PHP along with the stable phpzpk library to capture screenshots of a website.
If you are interested in other capture methods that do not require using an API, read to the end of the article for details on possible implementations of a daemon-type capture service, server requirements, and important security considerations.
Installing the Screenshot Capture Library
The phpzpk library incorporates all APIs from zpksystems, including HTMLToImage, which is the one we are interested in and will allow us to capture screenshots of HTML code we provide or an external URL.
The installation is simple and has no extra dependencies.
Although we could use the API via JSON requests from PHP using CURL, using the SDK has some advantages, such as saving network traffic when an error in our capture code can be detected locally.
Obtaining an API Key
First, head over to the zpk.systems website and sign up as a new user. No credit card is required, and you'll receive free credits to start developing. At the time of writing this article, the free credit is €2, which does not expire and allows you to make around 1,000 captures.
Remember, if you signed up using an email, the free credit won't be added until your email is validated.
Get API KeyCreating a Capture Application
The next step is to create an application. Click on "New Application" from your dashboard.
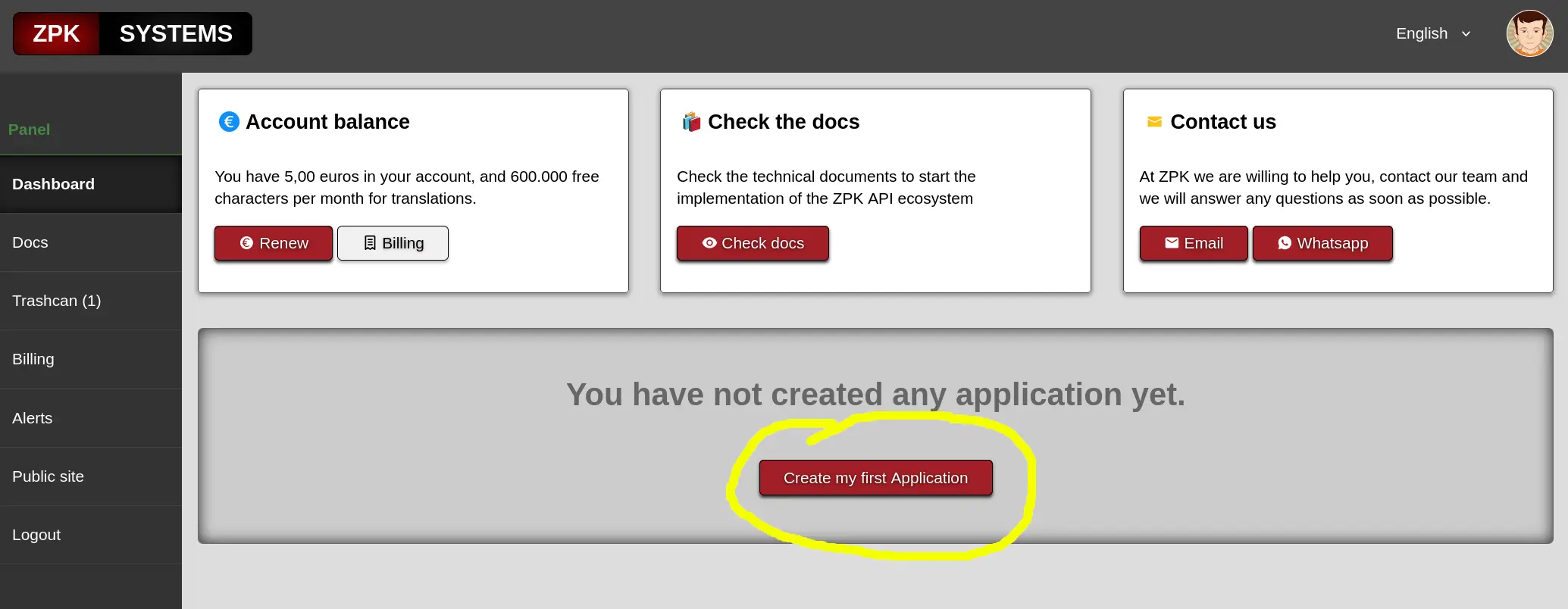
Give it a descriptive name, such as "Page Capture," and make sure the combo box is set to "Active Application."
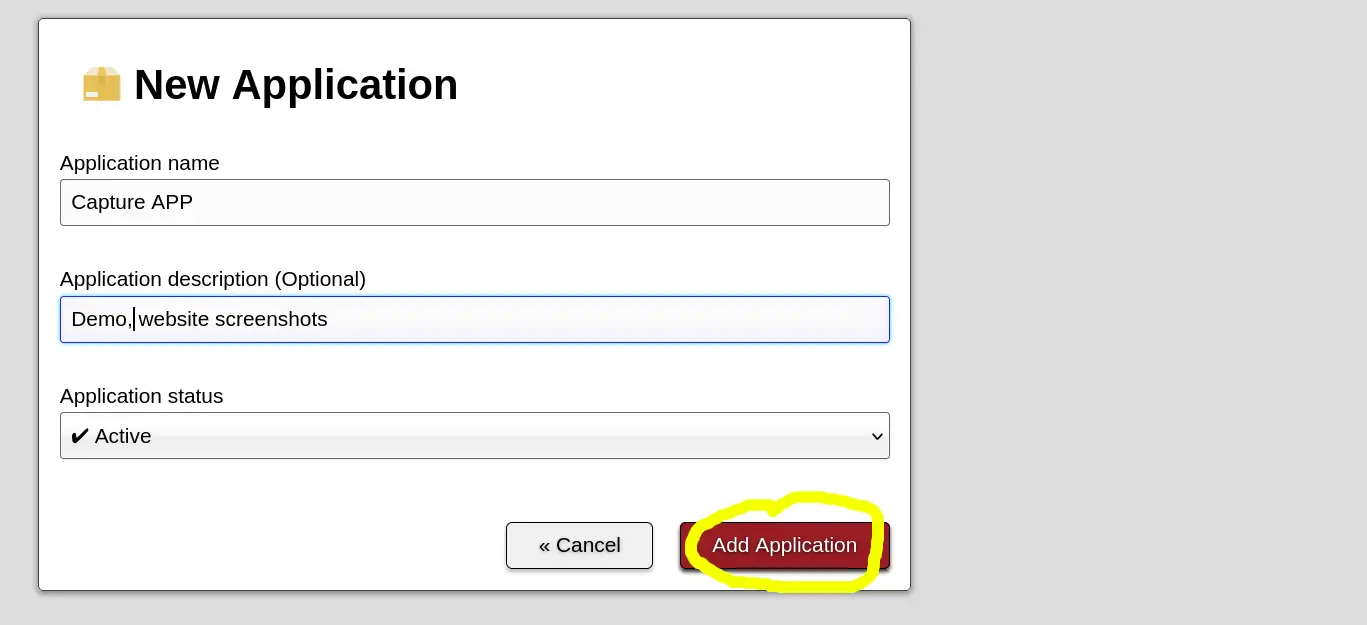
Once you do this, a confirmation window will appear with your capture application ID and API KEY. Make sure to copy both, as you'll need them for your PHP code.
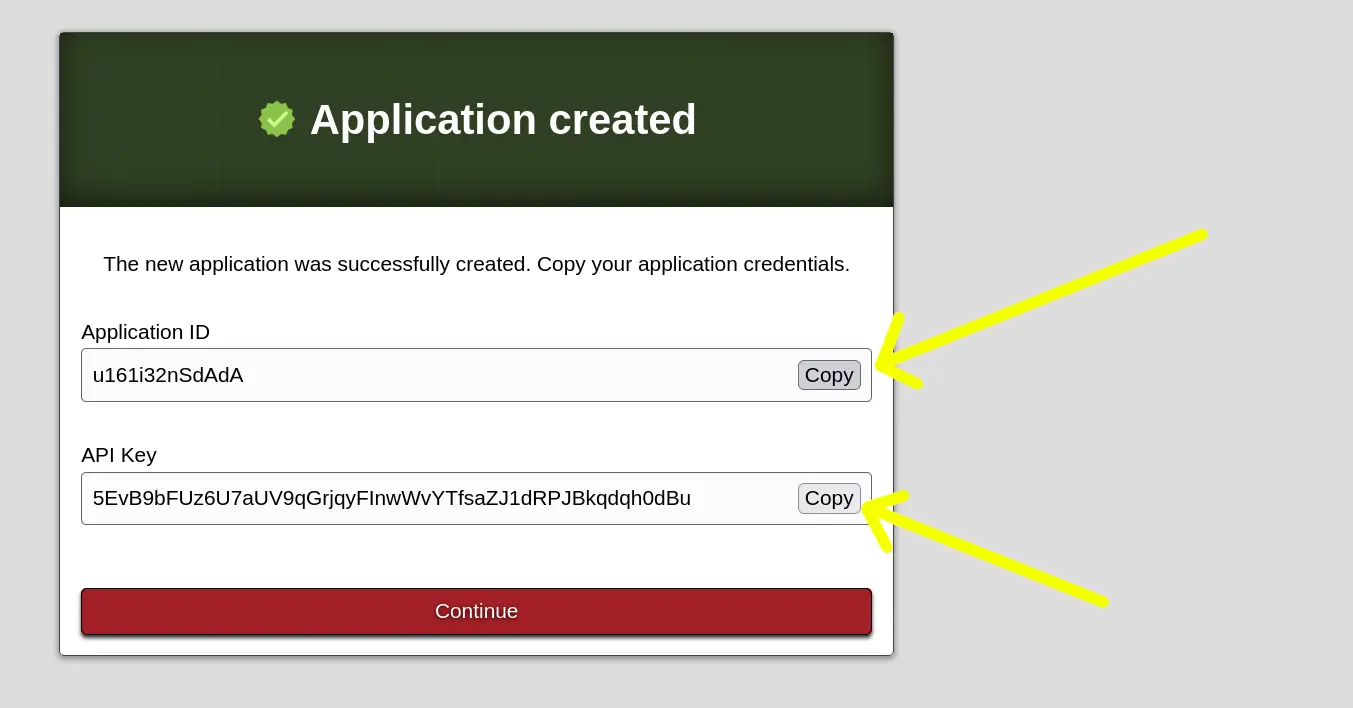
Basic Capture Code
As an example, we’ll capture this very article we’re reading.
First, we’ll instantiate the application we’re using by passing in our application ID and the API Key.
use zpksystems\phpzpk\zpkApplication;
use zpksystems\phpzpk\zpkHtmlToImageCapturer;
$app = new zpkApplication("APP_ID","API_KEY");
Now we’ll initialize a page capture by specifying the URL of the website we want to capture. We’ll also indicate that we want a high-resolution screenshot, suitable for desktop. If we prefer, we could lower the resolution to get a mobile version of a responsive page.
In this example, we’ll request a screenshot at a standard resolution of 1280x1024.
$url = 'https://zpk.systems/en/articles/article/howto-capture-website-screenshots-php';
$capturer = new zpkHtmlToImageCapturer($app,$url);
$capturer->setResolution(1280,1024);
The HtmlToImage library is designed to capture specific DOM elements (one or more). Since we want a screenshot of the entire page, we’ll simply set the HTML DOM element as the capture selector, meaning the whole document.
This capability to capture specific DOM elements is useful when we want to render HTML to an image, for example, to create our own components for article previews on social media. If you’re interested in this topic, here’s an article discussing the rendering of HTML components.
$el = $capturer->addElement('html');
Finally, we call the capture method, which requests the server to render our page and capture that element.
$capturer->capture();
It's important to note that we can capture up to five elements without increasing the cost of the API request.
As we can see in the output of our script, the API will return a list of the elements along with a boolean indicating whether the capture was successful.
If "success" returns true, it means the webpage was captured, and we will have a download URL for the image.
We can directly download that image using the download method of the HTML capture element we instantiated.
// Validamos que el elemento fué capturado
if( $el->isGenerated() ){
// Guardamos en un archivo
$el->download('/tmp/captura.png');
// O bien obtenemos el contenido directamente
// Para por ejemplo guardarlo en una base de datos.
$contenido = $el->getDownloadedContent();
}else{
echo "Ocurrio un error al capturar el elemento\n";
}
Complete Capture Script
The demonstration script is available on GitHub (website_screenshot_demo), so if you want to run a test, you only need to modify the APPLICATION_ID and the API key.
use zpksystems\phpzpk\zpkApplication;
use zpksystems\phpzpk\zpkHtmlToImageCapturer;
// Aplicación
$app = new zpkApplication("APP_ID","API_KEY");
$url = "https://zpk.systems/en/docs/api/all-apis";
// Inicializamos un capturador con nuesta app y
// establecemos la resolución (opcional)
$capturador = new zpkHtmlToImageCapturer($application,$url);
$capturador->setResolution(1280,1024);
$el = $capturer->addElement('html');
// Disparamos la captura del elemento HTML
echo "Realizando captura screenshot de $url... \n";
$response = $capturer->capture();
// Para ver la información retornada
print_r($response);
// Si se pudo capturar el elemento, mostramos URL de descarga,
// y descargamos en el archivo ejemplo.png
if( $el->isGenerated() ){
echo "URL de la captura: ".$el->getDownloadUrl();
$el->download('captura.png')
}else{
echo "No se pudo capturar el elemento.\n";
}
Other Use Cases
In addition to taking screenshots and capturing web pages, this type of API has other applications. For example, they can be used to render components like blog post headers, auto-generated images for social media, or elements for printing, such as business cards or brochures generated in HTML and CSS.